Reading sensor data from a Verisure home alarm system
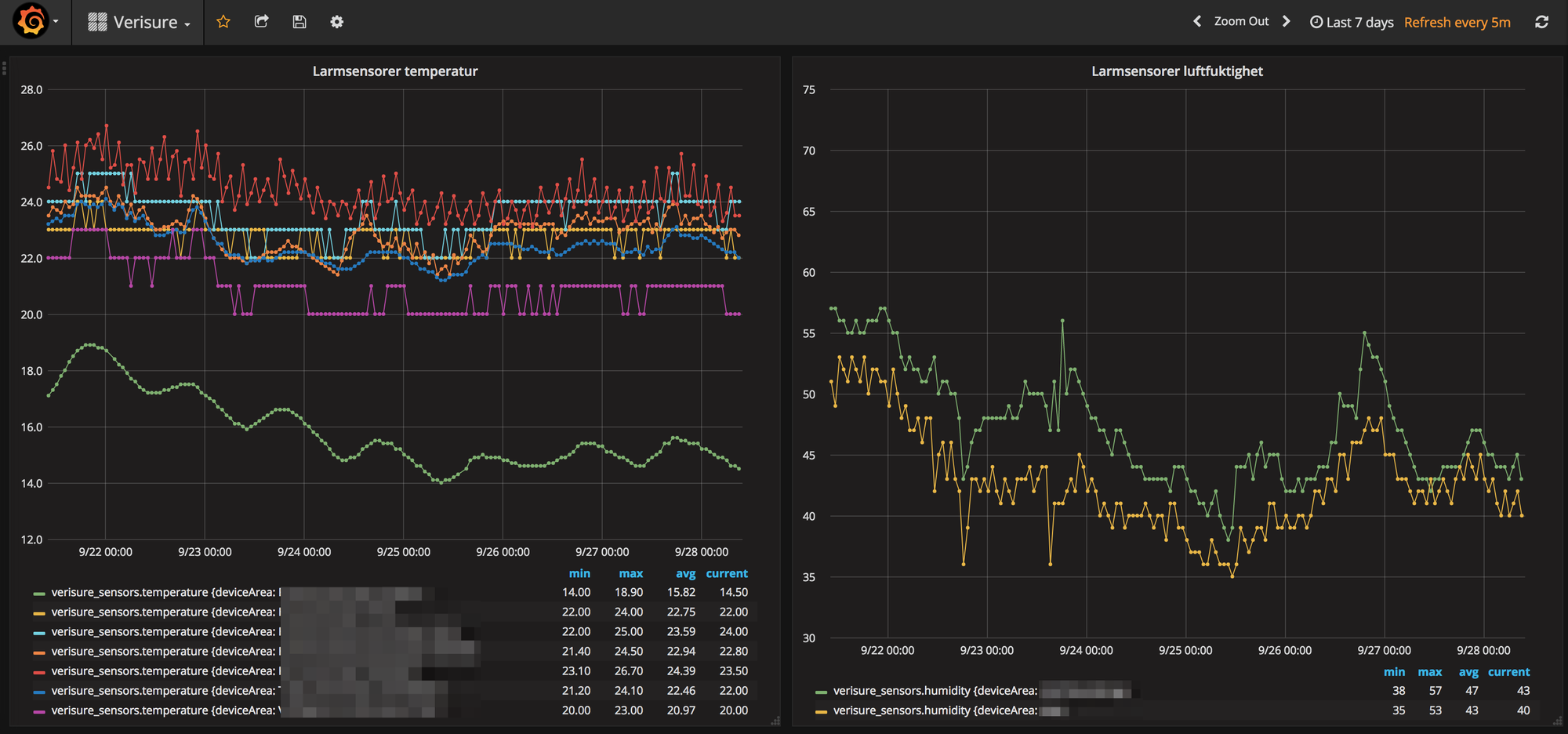
I recently upgraded our house alarm system to the latest model from Verisure.
Pretty nice actually, the new system comes with a good mobile app, remote querying of both alarm status and status of the various sensors (smoke, movement etc) that are included in the system. Oh, the new system actually cost less per month too, compared to the old one. Go figure.
Being the geek that I am, I immediately wondered if it was possible to get hold of the sensor readings (temperature and humidity) that were shown in the mobile app.
Turns out it is pretty simple. There is a REST API which can be queried, and from there you get back all kinds of status for the system. With that data nicely structured in a JSON it’s then a breeze to send the data to a MQTT pub-sub broker for later use by whatever system that might need it. Easy in theory.
Turns out this was easy in reality too.
Tech overview
First, the GitHub repository has a lot of tech oriented info. Please check it out.
A small Node.js app queries the API a few times per hour, then send the received data onward as MQTT messages.
This worked well, but for ease of deployment and configuration, a Docker setup would be even better.
A couple of Dockerfile and docker-compose.yml files took care of that, and the result is a stand-alone Docker container that provides quite useful environmental data, together with MQTT messages when someone arms/disarms the alarm system etc.
The MQTT topics are defined as follows:
<rootTopic>/verisure/various/topics/covering/different/data
The rootTopic is set in the docker-compose.yml file, see below.
Having the data available as MQTT messages is convenient, as it is then easy to use the data in other systems too.
MQTT topics with special meaning
<rootTopic>/status/services/verisure-to-mqtt-bridge
When the application starts up a “Hello mqtt” message will be sent to this topic.
<rootTopic>/verisure/tele/armState/STATE
This is the alarm’s current state, i.e. armed or not armed. This message is retained, i.e. it is available also for new MQTT clients that start subscribing to this topic.
To examine what MQTT topics the application uses, try subscribing to <rootTopic>/verisure/# from your favourite MQTT client. This will shown all messages generated by the application.
The source code of course tells all about what messaged are used.
Node-RED and Grafana
For example, here is a Node-RED flow that takes the MQTT messages, splits them up into individual sensor readings and then forward these to InfluxDB:
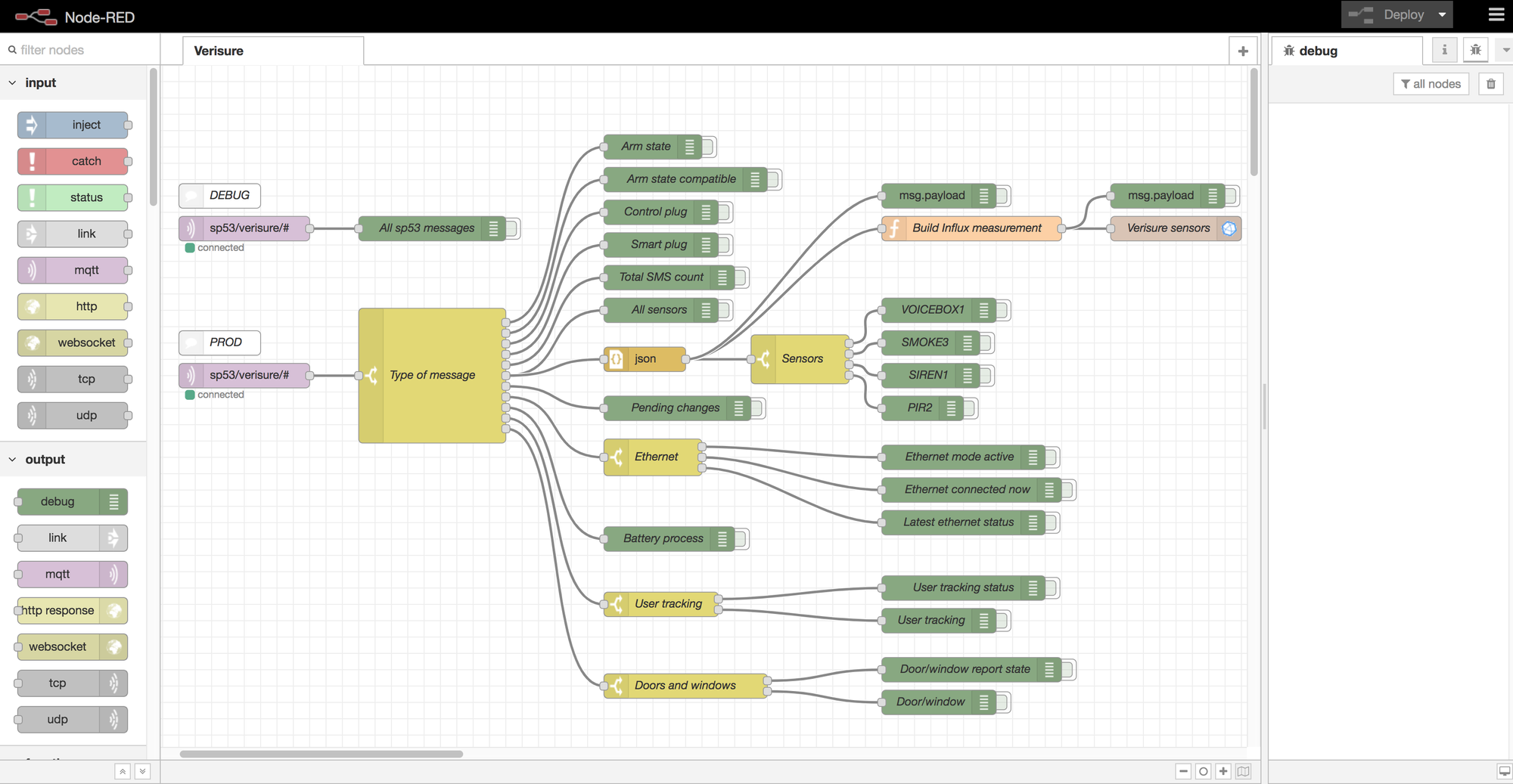
From there the data can be visualised in Grafana dashboards:
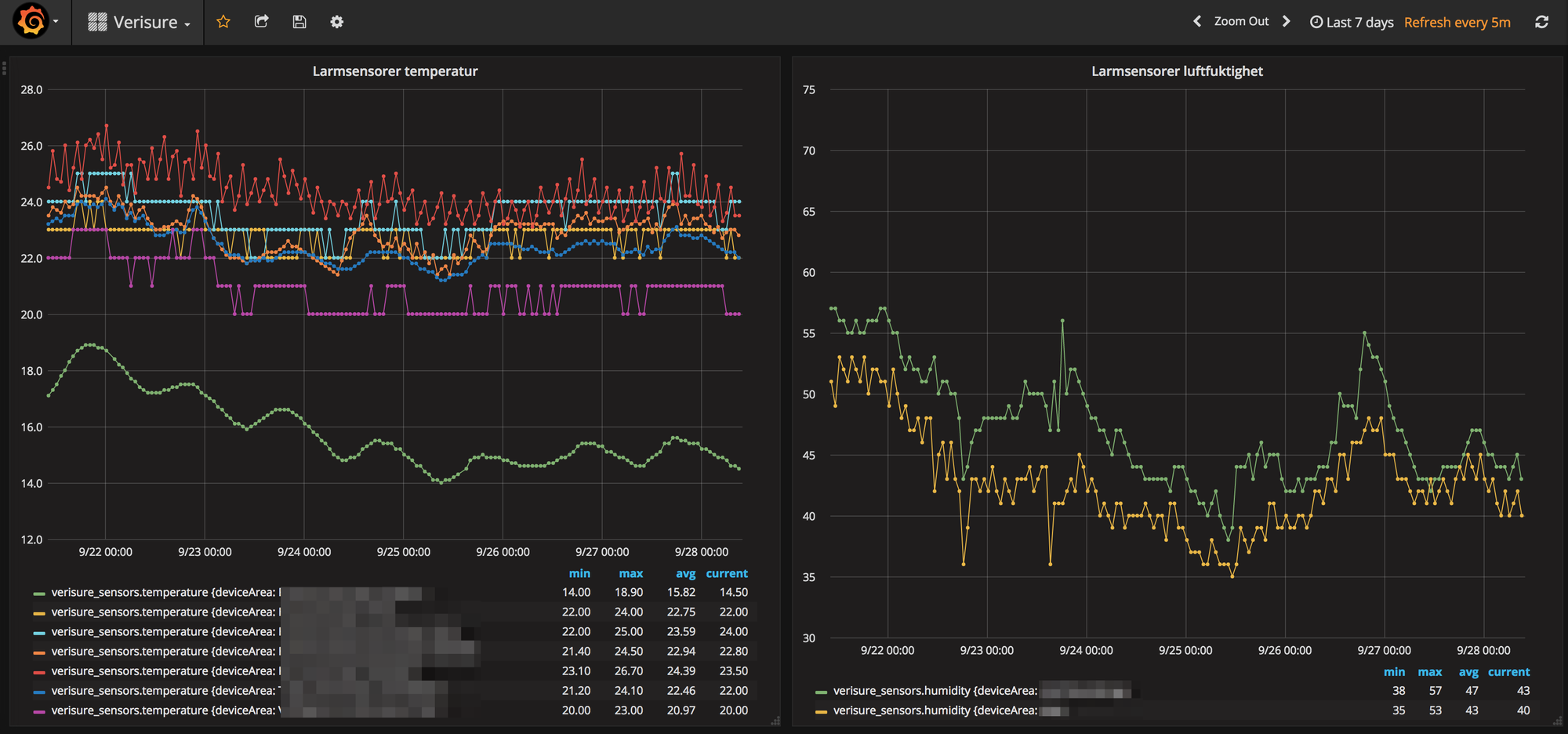
Configuration
All configuration is done in the docker-compose.yml file:
# docker-compose.yml
version: '2.2'
services:
verisure-mqtt:
# Use image on Docker Hub
image: mountaindude/verisure-mqtt:latest
init: true
container_name: verisure-mqtt
restart: always
# Set debug flag to true to output all received info to console.
# False will limit output to only mention that a query to the Verisure API is made
environment:
- "VERISURE_DEBUG=true"
- "MQTT_BROKER_HOST=<ip of MQTT broker>"
- "MQTT_BROKER_PORT=<port used by MQTT broker>"
- "MQTT_ROOT_TOPIC=myHouse/"
- "VERISURE_USERNAME=<username used to log into Verisure web service>"
- "VERISURE_PWD=<password used to log into Verisure web service"
logging:
driver: json-file
Place this file in an empty directory on the computer (with the Docker runtime available on it!), then edit the environment variables.
Run “docker-compose up” to pull down the Docker image from Docker Hub and start the container. If the VERISURE_DEBUG debug flag is set to true, all data received from the API calls will be output to the console. Useful for debugging.
“docker-compose up -d” will start the container in background mode.